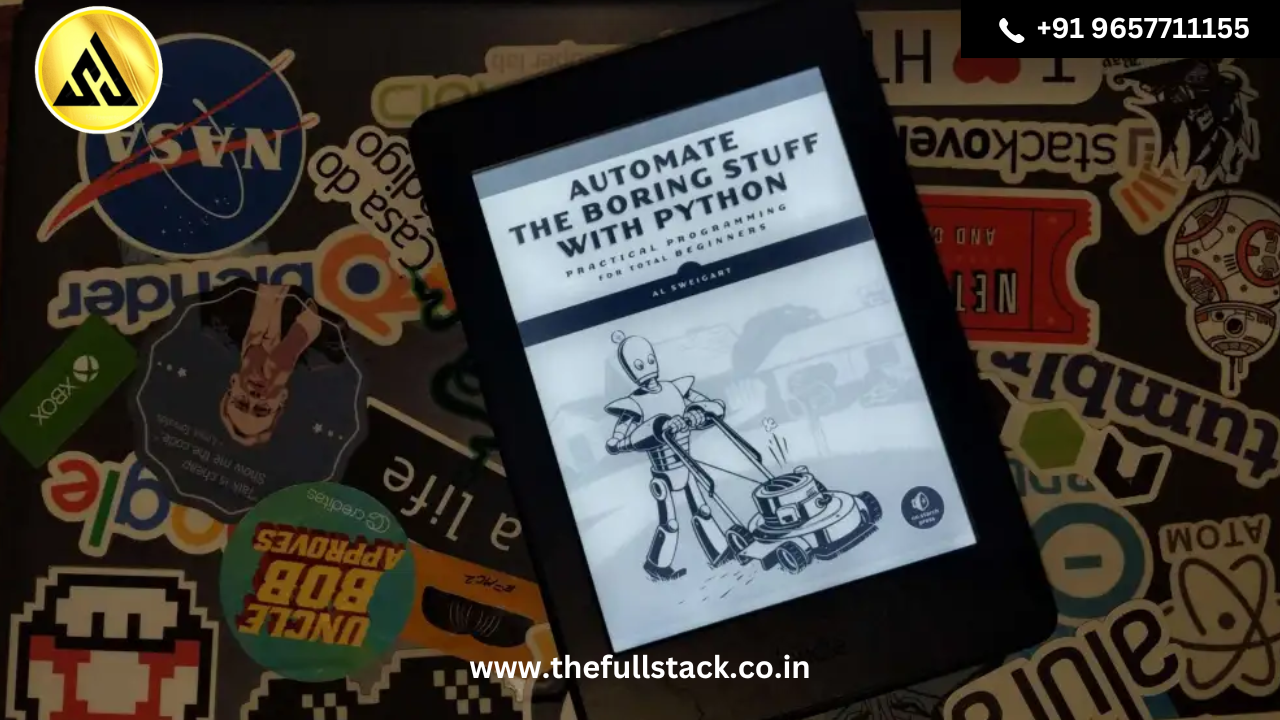
Automate Boring Stuff In Python-2024
Automate Boring Stuff In Python: Welcome to the world of Python, a powerful tool that transforms and simplifies tasks and opens a realm of possibilities for those willing to explore its depths.
In this blog post, we will guide you through Automating Boring Stuff with Python, showcasing how this versatile language can be your ally in navigating through the tedious tasks that consume your day, leaving room for creativity and innovation.
Python, with its simple syntax and vast libraries, stands as a beacon for both beginners and seasoned programmers. It democratizes the ability to automate routine tasks, from handling spreadsheets to parsing through web data. This introduction aims to provide you with practical advice, leveraging Python to not just enhance productivity but also to bring a sense of accomplishment in your daily work.
Drawing from the expertise of professionals who’ve mastered the art of automation, we’ll share insights that resonate with trust and clarity. By advocating a step-by-step approach, we make Python accessible, breaking down complex scripts into understandable segments that showcase the language’s power in making the impossible possible.
Also Read: Beautiful Soup In Python: BeautifulSoup Web Scraping Guide
Automate the Boring Stuff in Python
“Automate the Boring Stuff with Python” teaches programming using Python with a focus on practical, real-world automation tasks. The content covers various topics and projects that help users automate repetitive tasks and streamline workflows. Here are some key aspects and chapters covered in “Automate the Boring Stuff with Python”:
A) Introduction to Python
The “Introduction to Python” section in “Automate the Boring Stuff with Python” serves as the starting point for beginners to get acquainted with the Python programming language. It covers fundamental concepts and provides an overview of Python’s syntax and basic features. Here are key elements typically covered in an introduction to Python:
1) Python Basics:
Python is introduced as a high-level, interpreted programming language, known for its simplicity and readability. The syntax resembles the English language, making it beginner-friendly.
2) Setting Up Python:
The section provides step-by-step instructions for installing and setting up Python on different operating systems. This includes downloading the Python interpreter and, optionally, a code editor or integrated development environment (IDE) for writing and running Python code.
3) Running Python Code:
Learners are introduced to the interactive Python shell, where they can enter Python commands and see immediate results. Additionally, the concept of writing Python scripts in text editors or IDEs is explained.
4) Variables and Data Types:
The basics of variables are covered, emphasizing their role as containers for storing data. Common data types in Python, such as integers, floats, strings, and booleans, are introduced.
5) Basic Operations:
Fundamental arithmetic operations (addition, subtraction, multiplication, division) are explained. String concatenation and basic string manipulation are also introduced.
6) Printing Output:
The print() function is introduced as a way to display information to the console. Learners understand how to format and output different types of data.
7) Comments:
The use of comments in Python is explained. Comments are essential for adding explanatory notes within the code, enhancing code readability.
8) Indentation and Code Blocks:
Python’s unique indentation-based syntax is highlighted. The concept of code blocks defined by indentation is discussed, emphasizing the importance of consistent indentation for correct code execution.
9) Flow Control:
Control flow structures, such as if statements for conditional execution and loops (while loops and for loops) for repetitive tasks, are introduced. Learners understand how to control the flow of their programs based on conditions.
10) Functions:
– The basics of defining functions and calling them are covered. Functions are presented as a way to encapsulate and reuse code.
11) Error Handling:
– An introduction to error handling is provided, demonstrating the use of try-except blocks to gracefully handle errors that may occur during program execution.
B) Python Basics
Let’s delve into more detail about the Python Basics covered in the “Automate the Boring Stuff with Python”:
- Variables and Data Types:
Variables: A variable is a container for storing data values. In Python, you can create variables on-the-fly without specifying their data type. For example:
name = “John”
age = 25
height = 5.9
is_student = True
Data Types: Common data types include:
- Integers (int): Whole numbers without decimals.
- Floats (float): Numbers with decimals.
- Strings (str): Sequences of characters, enclosed in single or double quotes.
- Booleans (bool): True or False values.
- Basic Operations:
Python supports basic arithmetic operations:
addition_result = 5 + 3
subtraction_result = 7 – 2
multiplication_result = 4 * 6
division_result = 10 / 2
- String concatenation:
- python
- Copy code
greeting = “Hello”
name = “John”
full_greeting = greeting + ” ” + name
- Printing Output:
The print() function is used to display output in the console:
print(“Hello, World!”)
- Comments:
Comments start with the # symbol and are used for adding explanatory notes within the code:
# This is a single-line comment
- Indentation and Code Blocks:
Python uses indentation to define code blocks. Consistent indentation is crucial for proper code execution:
if condition:
# Code block with indentation
print(“Condition is True”)
- Flow Control:
If Statements: Used for conditional execution.
if x > 0:
print(“x is positive”)
elif x < 0:
print(“x is negative”)
else:
print(“x is zero”)
7) Loops:
While Loop: Executes a block of code while a condition is True.
while count < 5:
print(count)
count += 1
For Loop: Iterates over a sequence (e.g., a list or range).
for i in range(5):
print(i)
- Functions:
Functions are defined using the def keyword and can be called with arguments:
def greet(name):
print(“Hello, ” + name + “!”)
greet(“John”)
- Error Handling:
Try-except blocks are used for handling errors:
try:
result = 10 / 0
except ZeroDivisionError:
print(“Cannot divide by zero”)
C) Working with Strings:
- String Manipulation and Methods:
Concatenation: Combining strings using the + operator.
first_name = “John”
last_name = “Doe”
full_name = first_name + ” ” + last_name
String Methods: Python provides a variety of string methods for manipulation, such as:
sentence = “Hello, world!”
uppercase_sentence = sentence.upper()
lowercase_sentence = sentence.lower()
String Indexing and Slicing: Accessing individual characters or substrings within a string using indices.
word = “Python”
first_letter = word[0]
substring = word[1:4] # Returns ‘yth’
- Regular Expressions for Text Pattern Matching:
Regular Expressions (Regex): A powerful tool for matching patterns in text.
import re
text = “The phone number is 123-456-7890.”
pattern = re.compile(r’d{3}-d{3}-d{4}’)
match = pattern.search(text)
phone_number = match.group()
Common Regex Patterns:
- d: Matches any digit.
- w: Matches any alphanumeric character.
- .: Matches any character except a newline.
- *: Matches zero or more occurrences.
D) Handling Lists:
- Lists, Tuples, and Dictionaries:
Lists (list): Ordered and mutable collections of items.
fruits = [‘apple’, ‘banana’, ‘orange’]
Tuples (tuple): Ordered and immutable collections.
coordinates = (3, 4)
Dictionaries (dict): Unordered collections of key-value pairs.
person = {‘name’: ‘John’, ‘age’: 30, ‘city’: ‘New York’}
- Working with Data Structures:
- Accessing Elements: Using indices to access elements in lists and tuples.
first_fruit = fruits[0]
x_coordinate = coordinates[0]
- Dictionary Operations:
person[‘age’] = 31 # Updating a value
person[‘gender’] = ‘Male’ # Adding a new key-value pair
E) Automating Tasks:
- Reading and Writing Files:
with open(‘example.txt’, ‘r’) as file:
content = file.read()
with open(‘output.txt’, ‘w’) as file:
file.write(‘This is a new file.’)
- Organizing Files and Folders:
- File and Directory Operations:
import os
# Listing files in a directory
files = os.listdir(‘/path/to/directory’)
# Creating a new directory
- os.mkdir(‘new_directory’)
F) Web Scraping:
- Extracting Data from Websites using Web Scraping Techniques:
- HTML and DOM Structure: Understanding the structure of HTML (HyperText Markup Language) and the Document Object Model (DOM) is crucial for web scraping. Web pages are built using HTML, and the DOM represents the structure of the page in a hierarchical manner.
- HTTP Requests: Python’s requests library is employed to send HTTP requests to a website and retrieve the HTML content of web pages.
import requests
response = requests.get(‘
html_content = response.text
- Inspecting Elements: Browser Developer Tools or browser extensions are used to inspect the HTML structure of web pages and identify the elements containing the desired data.
- Introduction to Beautiful Soup and Requests Library:
- Beautiful Soup (bs4): A Python library for pulling data out of HTML and XML files. It provides Pythonic idioms for iterating, searching, and modifying the parse tree.
from bs4 import BeautifulSoup
soup = BeautifulSoup(html_content, ‘html.parser’)
- Navigating the Parse Tree: Beautiful Soup allows easy navigation of the HTML parse tree using methods like find(), find_all(), and attribute access.
title = soup.title
paragraphs = soup.find_all(‘p’)
G) Working with Excel Spreadsheets:
- Reading and Writing Excel Files:
- openpyxl Library: This library is used for reading and writing Excel files (XLSX format).
import openpyxl
# Reading from Excel file
wb = openpyxl.load_workbook(‘example.xlsx’)
sheet = wb[‘Sheet1’]
cell_value = sheet[‘A1’].value
# Writing to Excel file
new_wb = openpyxl.Workbook()
new_sheet = new_wb.active
new_sheet[‘A1’] = ‘Data’
new_wb.save(‘new_example.xlsx’)
- Manipulating Spreadsheet Data:
- Cell Operations: Reading and modifying cell values in Excel sheets.
cell_value = sheet.cell(row=1, column=1).value
sheet[‘A1’] = ‘New Data’
- Iterating Through Rows and Columns:
for row in sheet.iter_rows(min_row=1, max_row=3, min_col=1, max_col=3):
for cell in row:
print(cell.value)
H) Working with CSV Files:
- Processing Data in CSV Format:
- CSV (Comma-Separated Values): A common format for storing tabular data.
import csv
# Reading from CSV file
with open(‘example.csv’, ‘r’) as csv_file:
csv_reader = csv.reader(csv_file)
for row in csv_reader:
print(row)
with open(‘new_example.csv’, ‘w’, newline=”) as new_csv_file:
csv_writer = csv.writer(new_csv_file)
csv_writer.writerow([‘Name’, ‘Age’, ‘City’])
csv_writer.writerow([‘John’, ’30’, ‘New York’])
- CSV File Manipulation:
- Working with Rows and Columns: Similar to Excel, CSV operations involve reading and writing rows and columns of data.
with open(‘example.csv’, ‘r’) as csv_file:
csv_reader = csv.DictReader(csv_file)
for row in csv_reader:
print(row[‘Name’], row[‘Age’])
I) Working with JSON Data:
- Handling JSON Data in Python:
- JSON (JavaScript Object Notation): A lightweight data-interchange format. It is easy for humans to read and write and easy for machines to parse and generate.
- json Module: Python’s json module is used to handle JSON data.
import json
# Converting Python object to JSON
data = {‘name’: ‘John’, ‘age’: 30, ‘city’: ‘New York’}
json_data = json.dumps(data)
# Converting JSON to Python object
python_object = json.loads(json_data)
J) APIs and JSON:
- Introduction to APIs:
- API (Application Programming Interface): A set of rules that allows one software application to interact with another.
- RESTful APIs: Many web services and platforms expose APIs that follow RESTful principles, using HTTP methods (GET, POST, etc.) for communication.
- Making API Requests and Working with JSON Responses:
- requests Library: Used for making HTTP requests to interact with APIs.
import requests
# Making a GET request
response = requests.get(‘
# Working with JSON response
json_response = response.json()
K) Web Automation:
- Automating Web Browser Tasks using Selenium:
-
- Selenium: A powerful tool for controlling web browsers through programs and performing browser automation.
- WebDriver: Selenium requires a web driver to interact with browsers (e.g., ChromeDriver, GeckoDriver for Firefox).
- Automating Browser Interactions:
from selenium import webdriver
# Opening a browser window
driver = webdriver.Chrome()
# Navigating to a URL
driver.get(‘
# Interacting with elements
search_box = driver.find_element(‘css selector’, ‘input[name=”q”]’)
search_box.send_keys(‘Python automation’)
search_box.submit()
L) GUI Automation:
- Automating Graphical User Interface (GUI) Interactions:
- PyAutoGUI: A Python module that provides functions for programmatically controlling the mouse and keyboard.
- Automating Mouse and Keyboard Actions:
import pyautogui
# Moving the mouse
pyautogui.moveTo(100, 100)
# Clicking the mouse
pyautogui.click()
# Typing on the keyboard
- pyautogui.typewrite(‘Hello, World!’)
M) Debugging:
- Techniques for Debugging Python Code:
- Print Statements: Inserting print statements at various points in the code to output values and debug information.
def add_numbers(a, b):
print(f’Adding {a} and {b}’)
result = a + b
print(f’Result: {result}’)
return result
Using pdb (Python Debugger): Inserting breakpoints and running code in interactive mode.
import pdb
def divide(a, b):
pdb.set_trace() # Breakpoint
result = a / b
return result
- Inspecting Variables: Examining variable values during runtime to identify issues.
- Logging: Incorporating the logging module for more structured logging and debugging.
import logging
logging.basicConfig(level=logging.DEBUG)
logging.debug(‘Debugging information’)
N) Regular Expressions:
- In-Depth Exploration of Regular Expressions for Pattern Matching:
What Are Regular Expressions (Regex): Patterns used for matching character combinations in strings.
Regex Syntax:
- .: Matches any character except a newline.
- d: Matches any digit.
- w: Matches any alphanumeric character.
- *: Matches zero or more occurrences of the preceding element.
Using re Module:
import re
text = “The phone number is 123-456-7890.”
pattern = re.compile(r’d{3}-d{3}-d{4}’)
match = pattern.search(text)
phone_number = match.group()
O) Files and File Paths:
1) Reading from Files:
with open(‘example.txt’, ‘r’) as file:
content = file.read()
2) Writing to Files:
with open(‘output.txt’, ‘w’) as file:
file.write(‘This is a new file.’)
3) File Paths:
- os Module: Used for interacting with the operating system, including working with file paths.
import os
# Joining file paths
file_path = os.path.join(‘folder’, ‘subfolder’, ‘file.txt’)
# Getting the absolute path
absolute_path = os.path.abspath(‘example.txt’)
P) Debugging:
Strategies and Tools for Debugging Python Programs:
- IDE Debugging Tools: Integrated Development Environments (IDEs) often provide built-in debugging tools, including breakpoints, variable inspection, and step-by-step execution.
- Visual Studio Code Debugger: Using the debugger in Visual Studio Code for interactive debugging.
- PyCharm Debugger: PyCharm IDE offers a powerful debugger with features such as watches and expression evaluation.
Also Read: Beautifulsoup: Beginner’s Guide to Web Scraping in Python
Automate the Boring Stuff With Python
“Automate the Boring Stuff with Python” is a popular educational resource created by Al Sweigart. It serves as a comprehensive guide to learning Python programming through practical, real-world examples. The main focus of the material is to empower individuals to automate tedious and repetitive tasks using Python.
- Python Basics: The material starts with fundamental Python concepts, making it accessible for beginners with little to no programming experience.
- Working with Strings and Regular Expressions: Learners delve into manipulating text data, understanding string operations, and exploring regular expressions for pattern matching.
- Handling Files and Directories: The material covers file I/O operations, working with different file formats, and navigating directories using Python.
- Web Scraping and APIs: It introduces web scraping techniques to extract information from websites and explores working with APIs (Application Programming Interfaces) to interact with online services.
- Automating Tasks with Web and GUI Automation: Learners discover how to automate web browser tasks using tools like Selenium. Additionally, they explore GUI (Graphical User Interface) automation using PyAutoGUI.
- Debugging Techniques: The material provides insights into debugging Python code, including techniques such as print statements, using the Python Debugger (pdb), and utilizing logging.
“Automate the Boring Stuff with Python” is known for its hands-on approach, featuring practical exercises at the end of each chapter. The aim is to reinforce concepts and encourage learners to apply their skills to solve real-world problems. The material is freely accessible on the official website, making it an inclusive resource for anyone interested in automating tasks and enhancing their Python programming skills.
Whether you’re a beginner looking to enter the world of programming or an experienced developer seeking to streamline everyday tasks, “Automate the Boring Stuff with Python” offers valuable insights and practical knowledge for leveraging Python’s capabilities.
Automate Boring Stuff in Python FAQ’s
Is “Automate the Boring Stuff with Python” suitable for beginners?
Yes, the material is beginner-friendly. It assumes no prior programming experience and provides step-by-step instructions with examples. The goal is to empower beginners to automate tasks and solve real-world problems using Python.
Do I need any specific software or tools to follow along?
It primarily uses Python, and it is recommended using the Anaconda distribution, which includes Python and various scientific computing packages. Additionally, tools like a text editor or integrated development environment (IDE) are helpful for writing and running Python code.
Is this tutorial suitable for advanced Python programmers?
While the tutorial is beginner-friendly, it covers a variety of topics that may still be useful for intermediate and advanced Python programmers who want to expand their skills into automation, web scraping, and other practical applications.
What is “Automate the Boring Stuff with Python” about?
“Automate the Boring Stuff with Python” is a learning resource created by Al Sweigart that teaches practical Python programming skills for automating repetitive tasks, handling data, working with files, and more. The focus is on using Python to streamline everyday computer tasks.
Is the material freely accessible?
Yes, the material is freely accessible online. You can access it on the official website or through other online platforms.
you may be interested in this blog here:-
Advanced OOP Concepts in SAP ABAP A Comprehensive Guide
Leave a Reply