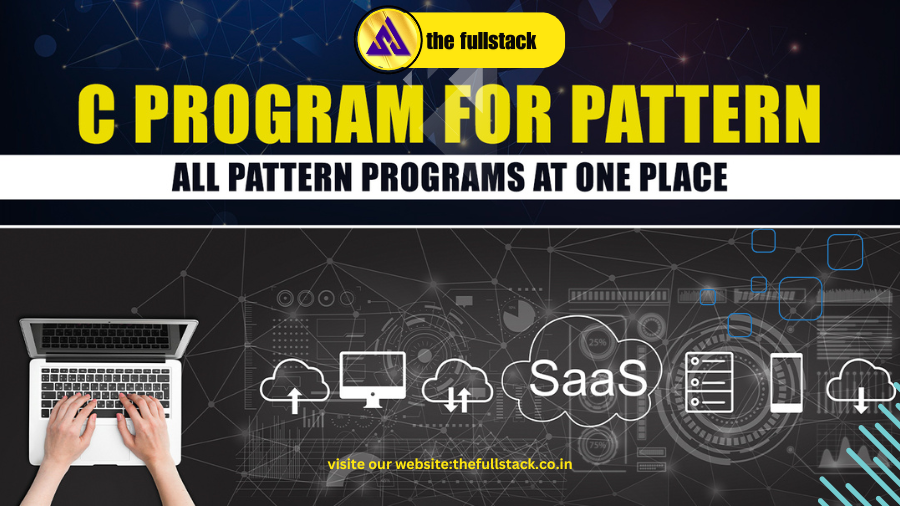
C Program For Pattern – All Pattern Programs At One Place-2024
So,
What Is C Program For Pattern?
A C program for pattern is a type of program written in the C programming language that generates various visual patterns using characters, numbers, or symbols. These programs commonly use loops, especially nested loops, to systematically print shapes such as stars, pyramids, rhombuses, and triangles on the screen. Writing pattern programs helps in understanding loop structures, enhances logical thinking, and is often a key part of technical interviews to test candidate’s coding skills and problem-solving abilities.
Types Of C Program For Pattern
We can use various types of C program to print differentC Program For Pattern. These patterns not only demonstrate your logical ability and technical skills but are also crucial for acing technical interviews. Understanding and practicing these patterns can surely enhance your coding proficiency and problem-solving capabilities.
In this article, we have compiled a detailed list of essential C program for pattern that are often asked in technical interviews. Each pattern comes with detailed explanations and code examples to help you understand the logic behind them. Below is a table listing the different types of pattern programs covered in this article:
Types Of C Program For Pattern |
|
By practicing these patterns, you’ll not only prepare for interviews but also enhance your overall programming skills.
1. Right Half Pyramid Pattern
In this pattern, stars are printed in a right-angled triangle format. The program uses two loops: the outer loop handles the number of rows, and the inner loop prints the stars in each row. The number of stars printed increases with each row. Below are the common steps that are being performed in this code.
- The user is asked to enter the number of rows.
- The outer loop (`for (i = 1; i <= rows; ++i)`) runs from 1 to the number of rows.
- The inner loop (`for (j = 1; j <= i; ++j)`) runs from 1 to the current row number, printing stars.
C Program For Pattern – Right Half Pyramid Pattern |
#include int main() { int i, j, rows;
printf(“Enter the number of rows: “); scanf(“%d”, &rows); for (i = 1; i <= rows; ++i) { for (j = 1; j <= i; ++j) { printf(“* “); } printf(“n”); } return 0; } |
Output- Enter the number of rows: 5*
* * * * * * * * * * * * * * |
2. Left Half Pyramid Pattern
In this pattern, stars are aligned to the right, forming a right-angled triangle against the right margin. This is achieved by first printing spaces, then stars. Below are the common steps that are being performed in this code.
- The user is asked to enter the number of rows.
- The outer loop (`for (i = 1; i <= rows; ++i)`) runs from 1 to the number of rows.
- The first inner loop (`for (j = i; j < rows; ++j)`) prints spaces to align stars to the right.
- The second inner loop (`for (j = 1; j <= i; ++j)`) prints stars.
C Program For Pattern – Left Hand Pyramid Pattern |
#include int main() { int i, j, rows;
printf(“Enter the number of rows: “); scanf(“%d”, &rows); for (i = 1; i <= rows; ++i) { for (j = i; j < rows; ++j) { printf(” “); } for (j = 1; j <= i; ++j) { printf(“* “); } printf(“n”); } return 0; } |
Output- Enter the number of rows: 5 *
* * * * * * * * * * * * * * |
3. Full Pyramid Pattern
The full pyramid pattern centers stars in a symmetrical triangle shape. The common steps involved in this process include-
- The user is asked to enter the number of rows.
- The outer loop (`for (i = 1; i <= rows; ++i)`) runs from 1 to the number of rows.
- The first inner loop (`for (j = i; j < rows; ++j)`) prints spaces to center the stars.
- The second inner loop (`for (j = 1; j <= (2 * i – 1); ++j)`) prints stars, forming a pyramid shape.
C Program For Pattern – Full Pyramid Pattern |
#include int main() { int i, j, rows;
printf(“Enter the number of rows: “); scanf(“%d”, &rows); for (i = 1; i <= rows; ++i) { for (j = i; j < rows; ++j) { printf(” “); } for (j = 1; j <= (2 * i – 1); ++j) { printf(“* “); } printf(“n”); } return 0; } |
Output- Enter the number of rows: 5 *
* * * * * * * * * * * * * * * * * * * * * * * * |
4. Inverted Right Half Pyramid Pattern
In this pattern, the maximum number of stars is in the first row, and it decreases with each row. The common step by step process involved in this program is written below for your reference.
- The user is asked to enter the number of rows.
- The outer loop (`for (i = rows; i >= 1; –i)`) runs from the number of rows down to 1.
- The inner loop (`for (j = 1; j <= i; ++j)`) runs from 1 to the current row number, printing stars.
C Program For Pattern – Inverted Right Half Pyramid |
#include int main() { int i, j, rows;
printf(“Enter the number of rows: “); scanf(“%d”, &rows); for (i = rows; i >= 1; –i) { for (j = 1; j <= i; ++j) { printf(“* “); } printf(“n”); } return 0; } |
Output-Enter the number of rows: 5* * * * *
* * * * * * * * * * |
5. Inverted Left Half Pyramid Pattern
This pattern aligns stars to the right margin, with spaces on the left. It starts with the maximum stars and decreases by one in each row. The common step by step process involved in this program is written below for your reference.
- The user is asked to enter the number of rows.
- The outer loop (`for (i = rows; i >= 1; –i)`) runs from the number of rows down to 1.
- The first inner loop (`for (j = i; j < rows; ++j)`) prints spaces to align stars to the right.
- The second inner loop (`for (j = 1; j <= i; ++j)`) prints stars.
C Program For Pattern – Inverted Left Half Pyramid |
#include int main() { int i, j, rows;
printf(“Enter the number of rows: “); scanf(“%d”, &rows); for (i = rows; i >= 1; –i) { for (j = i; j < rows; ++j) { printf(” “); } for (j = 1; j <= i; ++j) { printf(“* “); } printf(“n”); } return 0; } |
Output- Enter the number of rows: 5* * * * *
* * * * * * * * * * |
6. Inverted Full Pyramid Pattern
This program prints an inverted full pyramid pattern of stars, starting with the maximum number of stars at the top and decreasing the number of stars with each descending row. The pattern is symmetrical, with each row centered by spaces. The basic step-by-step process of this pattern is written below for your reference:
- Ask the user to enter the number of rows.
- Use an outer loop “for (i = rows; i >= 1; –i)” to iterate through the rows in descending order.
- Use the first inner loop “for (j = 0; j < rows – i; ++j)” to print spaces to align the stars centrally.
- Use the second inner loop to print stars for each row.
C Program For Pattern – Inverted Full Pyramid Pattern |
#include int main() { int i, j, rows;
printf(“Enter the number of rows: “); scanf(“%d”, &rows); for (i = rows; i >= 1; –i) { for (j = 0; j < rows – i; ++j) { printf(” “); } for (j = 1; j <= (2 * i – 1); ++j) { printf(“* “); } printf(“n”); } return 0; } |
Output- Enter the number of rows: 5* * * * * * * * *
* * * * * * * * * * * * * * * * |
7. Rhombus Pattern
This program prints a rhombus pattern of stars, where each side of the rhombus has an equal number of stars. The pattern is symmetric, with spaces used to align the stars correctly. The basic step-by-step process of this pattern is written below for your better :
- Ask the user to enter the number of rows.
- Use an outer loop “ for (i = 1; i <= rows; ++i)” to iterate through the rows.
- Use the first inner loop “ for (j = i; j < rows; ++j)” to print spaces for alignment.
- Use the second inner loop “for (j = 1; j <= rows; ++j)” to print stars for each row.
C Program For Pattern – Rhombus Pattern |
#include int main() { int i, j, rows;
printf(“Enter the number of rows: “); scanf(“%d”, &rows); for (i = 1; i <= rows; ++i) { for (j = i; j < rows; ++j) { printf(” “); } for (j = 1; j <= rows; ++j) { printf(“* “); } printf(“n”); } return 0; } |
Output- Enter the number of rows: 5 * * * * *
* * * * * * * * * * * * * * * * * * * * |
8. Diamond Pattern
This program prints a diamond pattern of stars. The diamond is symmetrical and centered, with an upper half that resembles a pyramid and a lower half that resembles an inverted pyramid. The basic step-by-step process of printing this pattern is written below for your better understanding:
- Ask the user to enter the number of rows.
- Use an outer loop to print the upper half of the diamond.
- Use the first inner loop to print spaces and the second inner loop to print stars.
- Use another outer loop to print the lower half of the diamond, following a similar logic.
C Program For Pattern – Diamond Pattern |
#include int main() { int i, j, rows;
printf(“Enter the number of rows: “); scanf(“%d”, &rows); //for printing the upper half of the diamond for (i = 1; i <= rows; ++i) { for (j = i; j < rows; ++j) { printf(” “); } for (j = 1; j <= (2 * i – 1); ++j) { printf(“* “); } printf(“n”); } // For printing the lower half of the diamond for (i = rows – 1; i >= 1; –i) { for (j = rows; j > i; –j) { printf(” “); } for (j = 1; j <= (2 * i – 1); ++j) { printf(“* “); } printf(“n”); } return 0; } |
Output-Enter the number of rows: 5 *
* * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * |
9. Hourglass Pattern
This program prints an hourglass pattern of stars. The pattern consists of an inverted full pyramid on top followed by a full pyramid at the bottom.
The basic step-by-step process of this pattern is written below for your reference:
- Ask the user to enter the number of rows.
- Use an outer loop to print the top inverted full pyramid.
- Use the first inner loop to print spaces and the second inner loop to print stars.
- Use another outer loop to print the bottom full pyramid, following a similar logic.
C Program For Pattern – Hourglass Pattern |
#include int main() { int i, j, rows;
printf(“Enter the number of rows: “); scanf(“%d”, &rows); // for printing upper inverted pyramid for (i = rows; i >= 1; –i) { for (j = 0; j < rows – i; ++j) { printf(” “); } for (j = 1; j <= (2 * i – 1); ++j) { printf(“* “); } printf(“n”); } // for printing lower pyramid for (i = 2; i <= rows; ++i) { //iteration from second row as the first row is taken from the inverted pyramid. for (j = i; j < rows; ++j) { printf(” “); } for (j = 1; j <= (2 * i – 1); ++j) { printf(“* “); } printf(“n”); } return 0; } |
Output- Enter the number of rows: 5* * * * * * * * *
* * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * |
10. Hollow Square Pattern
This program prints a hollow square pattern of stars. Only the border of the square is filled with stars, leaving the inside hollow. The basic step-by-step process of this pattern is written below for your reference:
- Ask the user to enter the size of the square.
- Use an outer loop to iterate through the rows.
- Use an inner loop to print stars on the border and spaces inside the square.
C Program For Pattern – Hollow Square Pattern |
#include int main() { int i, j, size;
printf(“Enter the size of the square: “); scanf(“%d”, &size); for (i = 1; i <= size; ++i) { for (j = 1; j <= size; ++j) { if (i == 1 || i == size || j == 1 || j == size) { printf(“* “); } else { printf(” “); } } printf(“n”); } return 0; } |
Output- Enter the size of the square: 5* * * * *
* * * * * * * * * * * |
11. Floyd’s Triangle Pattern
Floyd’s Triangle is a right-angled triangular array of natural numbers. It starts with 1 at the top left and increases sequentially row by row. This pattern is commonly used to test a programmer’s understanding of loops and sequences in programming. The basic step-by-step process of this pattern is written below for a better understanding of the concept:
- Ask the user to enter the number of rows.
- Use an outer loop to iterate through the rows.
- Use an inner loop to print numbers in each row.
- Use a counter to keep track of the current number.
C Program For Pattern – Floyd’s Triangle Pattern |
#include int main() { int i, j, rows, num = 1;
// Ask the user to enter the number of rows printf(“Enter the number of rows: “); scanf(“%d”, &rows); // Outer loop for each row for (i = 1; i <= rows; ++i) { // Inner loop for numbers in each row for (j = 1; j <= i; ++j) { printf(“%d “, num); ++num; // Increment the number } printf(“n”); // New line after each row } return 0; } |
Output- Enter the number of rows: 51
2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
So,
12. Pascal’s Triangle Pattern
This program prints Pascal’s Triangle, a triangular array where each number is the sum of the two directly above it. The general process of printing this pattern is written below for your reference:
- Ask the user to enter the number of rows.
- Use an outer loop to iterate through the rows.
- Use an inner loop to print the appropriate number of spaces for alignment.
- Use another inner loop to calculate and print the binomial coefficients.
C Program For Pattern – Pascal’s Triangle Pattern |
#include // Function to calculate factorial of a numberint factorial(int n) {
int fact = 1; for (int i = 1; i <= n; ++i) { fact *= i; } return fact; } int main() { int i, j, rows // Prompt user to enter the number of rows printf(“Enter the number of rows: “); scanf(“%d”, &rows); // Outer loop for each row for (i = 0; i < rows; ++i) { // Print leading spaces for alignment for (j = 0; j < rows – i – 1; ++j) { printf(” “); } // Inner loop to print binomial coefficients for (j = 0; j <= i; ++j) { printf(“%4d”, factorial(i) / (factorial(j) * factorial(i – j))); } printf(“n”); // New line after each row } return 0; } |
Output- Enter the number of rows: 5 1
1 1 1 2 1 1 3 3 1 1 4 6 4 1 |
Learn Programming With PW Skills
Are you looking for the perfect course to become a proficient programmer?
End your search here, as we are providing you with the best PW Skills C++ programming course, which is specially designed by experts considering various factors and demands of the users in mind. This beginner-friendly 6-month-long course comes with key features like training with expert mentors, skills lab and compiler for code practice, daily practice sessions, weekly coding competitions, job placement assistance, resume review sessions, and much more.
sequentially,So, what are you waiting for? Enroll today and start your journey of becoming a proficient programmer!
C Program For Pattern FAQs:
What is the purpose of learning pattern programs in C?
Pattern programs help in developing problem-solving skills, improve logical thinking, and enhance understanding of nested loops and control structures, which are essential for programming and technical interviews.
How do nested loops work in pattern programs?
In pattern programs, the outer loop usually controls the number of rows, while the inner loop manages the elements within each row, such as printing stars or spaces.
What is the importance of Floyd’s Triangle in C programming?
Floyd’s Triangle is a simple and effective way to practice sequential number printing and nested loop control, making it a good exercise for beginners.
Can pattern programs be extended to print numeric or alphabetic patterns?
Yes, patterns can be extended to print numbers, alphabets, or even custom characters by modifying the print statements and the values within the loops.
Leave a Reply