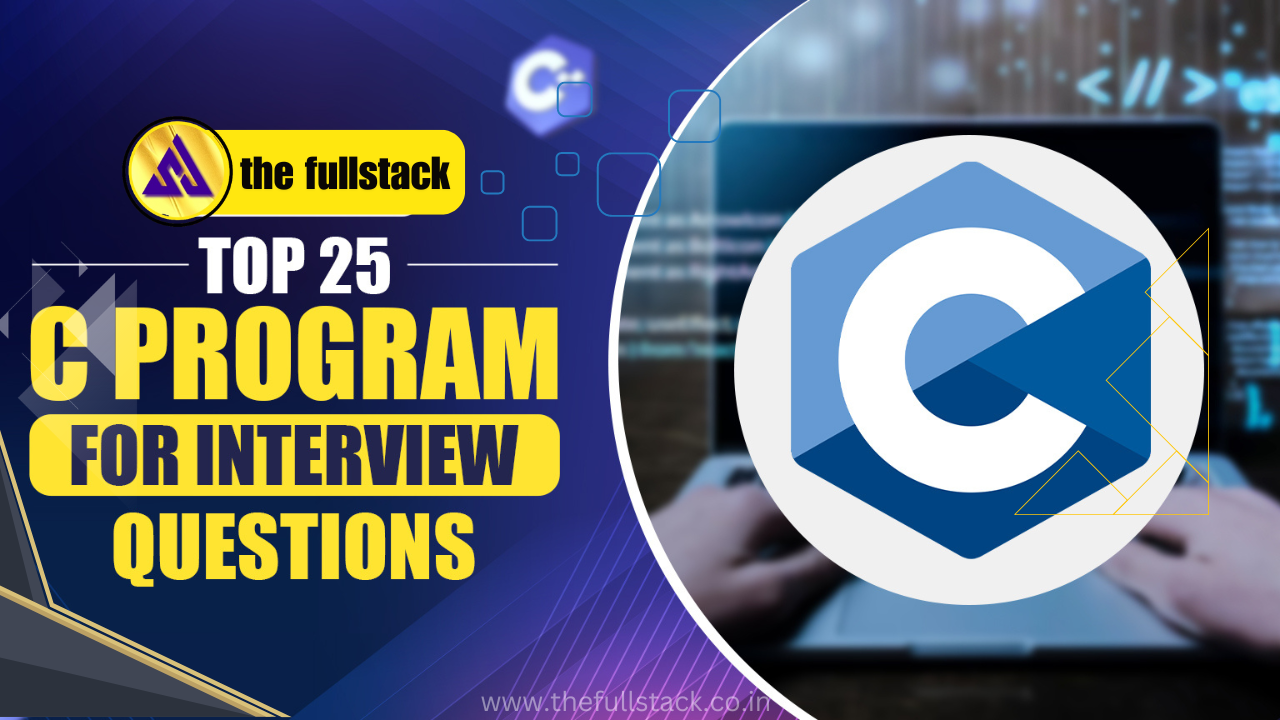
Top 30 Most Basic Programming Questions During Interviews-2024
In today’s competitive era, where finding a job is no less than a task, learning C Basic Programming Questions During Interviews can be a good choice that will help you stand out from the crowd. But the common question is what to learn and where to learn from. This article is designed to address this common concern.
After thorough research, We’ve compiled the top 30 C program for interview questions to guide you through the essential concepts and coding problems that are often asked in technical interviews. Whether you’re a beginner or looking to brush up on your skills, these questions will provide you with a solid foundation and boost your confidence as you prepare for your next big opportunity.
1 C Program For Interview Questions
C programming language is both challenging and essential for enhancing your programming skills. C Program for interview questions can be divided into two categories:
- For Freshers
- For Experienced Professionals
In this article, you’ll find the most frequently asked C programming interview questions and their answers for both fresher and experienced levels. These questions are specially picked by experts after researching the current market trends and interview patterns of various MNCs.
2 C Program For Interview Question – Fresher Level
Let us start with the interview question of the fresher level and then we will move forward to the experienced one.
Q1. Why is C called a mid-level programming language?
Ans) C is termed as a mid-level programming language because it bridges the gap between high-level languages and low-level languages. It combines the features of both, High-level languages are user-friendly and abstract away hardware details, while low-level languages are closer to machine code, providing more control over hardware.
C offers a balance of both by direct manipulation of hardware through pointers and memory addresses, while still supporting high-level constructs like functions and complex data types. This flexibility makes C language powerful for system programming, such as developing operating systems and embedded systems, while still being suitable for developing applications.
Q2. What are the features of the C programming language?
Ans) C programming language has several key features that contribute to its popularity and wide usability, some of the common key features of C language include-
- Simplicity: C has a straightforward syntax that is easy to learn and understand.
- Efficiency: Programs written in C are highly efficient and can run quickly, making it ideal for system-level programming.
- Portability: C programs can be easily transferred from one machine to another with minimal or no modification.
- Rich Library: C provides a rich set of built-in functions and libraries that simplify complex programming tasks.
- Extensibility: C allows programmers to add their own functions to the library, making the language highly extensible.
- Structured Language: C supports structured programming approach, that help organizing code into reusable modules.
- Low-level Manipulation: C provides low-level access to memory through pointers, making it suitable for tasks like – developing operating systems.
Q3. What are basic data types supported in the C Programming Language?
Ans) C supports several basic data types that are an essential part of programming in the language, These data types are the building blocks for data manipulation in C and are crucial for declaring variables, constants, and functions. some basic data types include:
Data Types | Uses |
Int | Used for storing integer values. |
Float | It is used for storing decimal numbers. For eg – Pie=3.14 |
Double | It is used to store double-precision decimal numbers. For Eg – 34.886242 |
Char | Used for characters. |
Void | It represents the absence of type. It is mainly used for functions that do not return any value |
Q4. What are tokens in C?
Ans) Tokens are the smallest elements of a program in C and are the building blocks of a C program. Understanding tokens is crucial as they form the syntax and semantics of the language. The basic six types of tokens in C are listed below for your reference:
Tokens | Uses |
Keyword | These are reserved words that have a special meaning in C, such as int, return, void. |
Identifiers | These include names given to variables, functions, arrays, etc., such as main, sum, i |
Constants | Fixed values that do not change during the execution of a program, like 10, 3.14. |
Operators | Symbols that perform operations on variables and values, such as “+”, “-”, and “*”. |
Special characters | Punctuation marks and symbols that have specific meanings, such as “{}”, “[]”, “;” |
Strings | Sequence of characters enclosed in double quotes, like “Hello, World!”. |
Q5. What do you mean by the scope of the variable?
Ans) The scope of a variable in C refers to the region of the program where the variable is accessible and can be used. There are mainly three types of variable scope:
- Local Scope: Variables declared within a function or block are accessible only within that function or block.
- Global Scope: Variables declared outside all functions are accessible from any function within the program.
- Block Scope: Variables declared inside a block denoted by `{}` are accessible only within that block.
Scope rules determine the visibility and lifetime of variables, which helps in managing memory and avoiding conflicts in variable names across different parts of the program.
Q6. What are preprocessor directives in C?
Ans) Preprocessor directives are commands that are processed by the C preprocessor before the actual compilation of the code begins. They provide instructions for the compiler to preprocess the source code. Preprocessor directives begin with the `#` symbol and include the common types listed below:
Preprocessor Directives | Uses |
#Define | It is used to define macros or constants. For example, `#define PI 3.14`. |
#Include | It is used to include the contents of a file or a library. For example, `#include `. |
#if, #ifdef, #elif, #endif | These are conditional compilation directives that allow inclusion or exclusion of parts of code based on certain conditions. |
#Undef | It is used to undefine a macro. |
#Pragma | It is used to provide special commands to the compiler. |
Q7. What is the use of static variables in C?
Ans) Static variables in C have a property of preserving their value even after they go out of scope. They are declared with the keyword `static`. The primary uses of static variables are:
- Maintaining State: In functions, static variables can regain their value between multiple function calls. For example, a static counter inside a function that counts the number of times the function is called.
- Scope Limitation: Static variables within a file have internal linkage, meaning they are not accessible from other files. This basically helps in preventing name conflicts.
- Efficient Memory Usage: Static variables are stored in the data segment of the memory, which reduces memory consumption as they do not require reallocation or reinitialization every time they are accessed.
- Static variables are useful for tasks that require persistent state information without using global variables.
Q8. What is the difference between malloc() and calloc() in C?
Ans) The basic difference between these two term are written below for your reference, reading the below table will clear all your doubts.
malloc() | calloc() |
malloc() is a function that creates one block of memory of a fixed size. | calloc() is a function that assigns a specified number of blocks of memory to a single variable. |
malloc() only takes one argument | calloc() takes two arguments. |
malloc() is faster than calloc. | calloc() is slower than malloc() |
malloc() has high time efficiency | calloc() has low time efficiency |
malloc() is used to indicate memory allocation | calloc() is used to indicate contiguous memory allocation |
malloc() does not initialize the memory to zero | calloc() initializes the memory to zero |
malloc() does not add any extra memory overhead | calloc() adds some extra memory overhead |
Q9. What do you mean by dangling pointers?
Ans) A dangling pointer in C is a pointer that stores a memory location that has already been freed or deallocated. This can lead to undefined behavior, including program crashes or corruption of data. Dangling pointers generally occur in the following scenarios:
- After Memory Deallocation: When memory allocated dynamically is freed using `free()`, but the pointer still points to that memory location.
- Function Return: When a function returns a pointer to a local variable, the pointer points to memory that is no longer valid once the function exits.
- Out of Scope: When a pointer points to a variable that goes out of scope.
To prevent dangling pointers, it’s good practice to set pointers to `NULL` after freeing the allocated memory or ensure that pointers are not used after the referenced memory is deallocated.
Q10. Write a program to convert a number to a string with the help of sprintf() function
Here is a simple C program to convert an integer number to a string using the `sprintf()` function:
C Program For Interview – Converting An Integer Into String Using Sprintf() |
#include int main() { int number = 12345;
char str[20]; // Using sprintf() to convert integer to string sprintf(str, “%d”, number); // Output the converted string printf(“The number as a string is: %s\n”, str); return 0; } |
Output- The number as a string is: 12345 |
In this program, the integer `number` is converted to a string using `sprintf()`, which formats the integer and stores it in the character array “str”. The formatted string is then printed using `printf()`function. The `sprintf()` function is flexible and can be used to convert different data types to strings.
Q11. What is recursion in C?
Ans) Recursion in C is a programming technique where a function calls itself directly or indirectly to solve a problem. This method is particularly useful for problems that can be broken down into smaller, similar subproblems. Each recursive call reduces the complexity of the problem and works towards a base case.
Q12. What is the difference between local and global variables in C?
Ans) Local variables help in managing memory efficiently and avoid unplanned modifications, while global variables provides facility of sharing data across multiple functions. Let us understand the common differences between these two terms with the help of the table below.
Local Variable | Global Variable |
Accessible only within the block of code where they are declared. | Accessible from any function in the program |
Exists only during the execution of the function/block. | Exists for the entire lifetime of the program. |
Declared within a function or block. | Declared outside of all functions. |
Must be explicitly initialized. | Automatically initialized to zero if not initialized. |
It is stored in the stack | It is stored in the data segment |
Q13. What are pointers and their uses?
Ans) Pointers are variables that store memory addresses of other variables. They are declared using the `*` operator and must be used carefully to avoid issues like dangling pointers and memory leaks. An example of the basic implementation of pointer is given below for your reference-
Example Of Pointer |
int *ptr;int var = 10;ptr = &var; // ptr now holds the address of var |
Basic uses of Pointers include:
- Pointers are used in accessing functions like `malloc` and `calloc`.
- Using pointers we can efficiently manage and manipulate arrays and strings.
- Pass large structures or arrays by reference rather than by value, which saves memory and processing time.
- It is used to implement complex data structures like linked lists, trees, and graphs.
- Pointers are used for accessing and manipulating memory locations.
Q14. What is typedef in C?
Ans) `typedef` in C is a keyword used to create new names for existing data types. This can simplify complex declarations and improve code readability. The common uses of typedef keywords include-
- It generally makes complex type declarations easier to understand.
- It provides a facility for easier code maintenance and portability by allowing changes to data type definitions without modifying the entire codebase.
- It is used for improving the clarity of code, especially when using complex structures and pointers.
Q15. What are loops and how can we create an infinite loop in C?
Ans) Loops in C are constructs that allow repeating a block of code multiple times. The main types of loops include for loop, while loop, and do-while loop. Examples of each type of loop are given below for your better understanding of the concept-
- For Loop:
A for loop is used to repeat a block of code a specific number of times. It has a fixed value for iteration, a condition, and an increment/decrement statement.
For Loop – C Program For Interview Questions |
for (int i = 0; i < 10; i++) { printf(“%d\n”, i);} |
- While Loop:
A while loop keeps executing a block of code as long as a specified condition is true. It’s useful when the number of iterations isn’t known priorly, and the loop will generally not run at all if the condition is initially false.
While Loop – C Program For Interview Questions |
int i = 0;while (i < 10) { printf(“%d\n”, i);
i++; } |
- Do-While Loop:
A do-while loop is similar to a while loop but the main difference is that it guarantees at least one execution of the loop body. The condition is checked after the block of code runs, ensuring that the code loop should run at least once.
Do-While Loop – C Program For Interview Questions |
int i = 0;do { printf(“%d\n”, i);
i++; } while (i < 10); |
Creating an Infinite Loop:
Infinite loops run indefinitely until an external condition breaks the loop. Here are examples of infinite loops using different types of loops.:
C Program For Interview Questions – Creating An Infinite Loop |
// using for, while, do-while loops#include int main()
{ for (;;) { printf(“Infinite-loop PW Skills\n”); } while (1) { printf(“Infinite-loop PW Skills\n”); } do { printf(“Infinite-loop PW Skills\n”); } while (1); return 0; } |
Infinite loops are useful in scenarios like continuously monitoring inputs, servers waiting for requests or real-time systems. However, they should always include a mechanism to break out of the loop to prevent the program from hanging.
Q16. What is the difference between type casting and type conversion?
Ans) Type casting and type conversion both involve converting a variable from one data type to another, but the difference between them is in how they are performed and their explicitness, let us understand their differences in detail with the help of the table given below.
Type Casting | Type Conversion |
It involves explicitly converting one data type to another using casting operators | It involves implicitly converting one data type to another by the compiler |
The programmer has full control over the conversion. | It is handled by the compiler based on predefined rules |
It is used when precise control over the conversion is needed. | 1-It is used when the conversion is straightforward and safe. |
It may lead to loss of data or precision if not done carefully | Generally safer as it follows predefined rules |
Type casting is useful when you need particular conversions, like truncating a float to an int, etc. | Type conversion simplifies code by automatically converting compatible types. |
Q17. What are header files and their uses?
Ans) Header files in C, basically ends with the extension `.h`. It contain declarations for functions, macros, constants, and data types used in multiple source files. They help in organizing code and provides features of reusability and modularity.
Uses of Header Files:
- It is used to declare functions used across different files.
- It also defines macros for constants and inline functions to improve code readability and maintenance.
- It is used for declaring custom data types, like `struct` and `enum`, for use across multiple files.
- Include standard library functions, like `stdio.h` for input/output operations and `stdlib.h` for utility functions.
18. What are the functions and their types?
Ans) Functions in C are blocks of code designed to perform specific tasks. They allow for code reuse, better organization, and easier debugging. Different types of functions include
- Built In Function: These are also called library functions, they are predefined functions provided by C standard libraries, like `printf()`, `scanf()`, `malloc()`.
- User-Defined Functions: These are custom functions created by programmers to perform specific tasks.
19. What is the difference between macro and functions?
Ans) Macros and functions in C serve different purposes and have different characteristics, some common differences in both of them are written below for your reference.
Macro | Function |
Macros are preprocessed. | Functions are compiled. |
Macro is used for increasing code length. | Using functions doesn’t usually affect code length. |
Using Macro leads to faster execution speed. | Execution speed is slower in functions. |
The macro name is replaced by the macro value before compilation. | Transfer of control takes place during the function call. |
Macro doesn’t check any Compile-Time Errors. | Function check Compile-time errors. |
20. What are reserved keywords?
Ans) Reserved keywords in C are words that have special meaning and purpose in the language. These keywords are reserved by the language and cannot be used as identifiers, variable names, function names, etc. in a program. These keywords are used for forming the structure of C program. Examples of these reserved keywords include – `int`, `return`, `if`, `else`, `while`, `for`, `switch`, `break`, `continue`, and `struct`.
Q21. What is a structure?
Ans) A structure in C is a user-defined data type that allows the grouping of variables of different types under a single name. It is defined using the `struct` keyword and is used to represent a record.
Below is the example of C program to implement Structure-
C Program For Interview Questions – Structure Implementation |
#include // Define a structurestruct Person {
char name[50]; int age; float salary; }; int main() { // Declare a structure variable struct Person person1; // Assign values to the structure members strcpy(person1.name, “Physics Wallah”); person1.age = 22; person1.salary = 55000.50; // Access and print the structure members printf(“Name: %s\n”, person1.name); printf(“Age: %d\n”, person1.age); printf(“Salary: %.2f\n”, person1.salary); return 0; } |
Output-Name: Physics WallahAge: 22
Salary: 55000.50 |
In this example, the `Person` structure groups variables `name`, `age`, and `salary`. Structures are useful for organizing complex data and creating custom data types that have less complexity.
Q22. What is a union?
Ans) A union in C is a user-defined data type similar to a structure, but with one key difference: all members of a union share the same memory location. This means that only one member can hold a value at any given time. Unions are defined using the `union` keyword.
The union can be useful in many situations where we want to use the same memory for two or more members. The basic example of Union is given below for your better understanding and clarity of concept.
C Program For Interview Questions – Structure Of Union |
#include // Define a unionunion Data {
int i; float f; char str[20]; }; int main() { // Declare a union variable union Data data; // Assign and access different members of the union data.i = 10; printf(“data.i: %d\n”, data.i); data.f = 220.5; printf(“data.f: %.2f\n”, data.f); strcpy(data.str, “Physics Wallah”); printf(“data.str: %s\n”, data.str); return 0; } |
Output-data.i: 10data.f: 220.50
data.str: Physics Wallah |
In this example, the `Data` union can store an integer, a float, or a string, but can only access one at a time. Unions are useful for memory-efficient storage of variables when only one value is needed at a time.
Q23. What are an r-value and l-value?
Ans) l-value: l-value is known as locator value, it basically represents an object that occupies some identified location in memory. l-values can appear on the left or right side of an assignment operator. We have written an example of l-operator below that will help you to understand it in better way.
Examples Of L-Value |
int x = 5; // x is an l-valueint *p = &x; // p is an l-value*p = 10; // *p is an l-value |
r-value: r-value is known as read value, It represents a value that does not occupy any identifiable memory location. r-values can only appear on the right side of an assignment operator. The basic example of r-value is written below for your better understanding.
Examples Of R-Value |
int y = 20; // 20 is an r-valuey = x + 5; // x + 5 is an r-value |
Q24. What is the difference between call by value and call by reference?
Ans) Call by value and call by reference, these two terms might feel alike from the name but they have different functions and tasks to perform. The common differences between these two terms are written below for your reference.
Call by value | Call by Reference |
The Values of the variable are passed when the function calls. | The location of the variable is passed when the function calls. |
Dummy variables generally copy the value of each variable in the function call. | In this case, Dummy variables copy the location of actual variables. |
The actual variable is not affected by the changes made to dummy variables | The address can be used here to manipulate actual variables. |
Values of the variables are passed using simple techniques. | Pointer variables are used here to store address of variables. |
Q25. What is the sleep() function?
Ans) The sleep() function in C is used to suspend the execution of the program for a specified number of seconds. sleep() function will basically sleep the current executable task for the specified amount of time but other operations of the CPU will function properly. It is defined in the `unistd.h` header file in Unix-based systems and the `windows.h` header file in Windows systems.
The sleep() function is useful for introducing delays in the execution of a program, for example – It is used for scenarios where we have to wait for a resource to become available or to create timed events.
Q26. What are Enumerations?
Ans) Enumerations commonly known as enums in C are user-defined data types that consist of integral constants. They enhance code readability by assigning meaningful names to sets of numeric values. Enumerations are declared using the `enum` keyword. For example, different days of the week can be defined as an enumeration and can be used anywhere in the program.
C Program For Interview Questions – Example Of Enumeration |
#include // Define an enumerationenum Day { Sunday, Monday, Tuesday, Wednesday, Thursday, Friday, Saturday };
int main() { enum Day today; today = Wednesday; printf(“Today is day number %d of the week.\n”, today); return 0; } |
In this example, the `Day` enum assigns meaningful names to the days of the week, with `Sunday` being 0, `Monday` being 1, and so on. Enumerations improve code clarity and reduce the chances of errors.
Q27. What is a volatile keyword?
Ans) The `volatile` keyword in C is used to inform the compiler that a variable’s value may be changed unexpectedly, outside the normal program flow. This prevents the compiler from optimizing code that assumes the variable’s value remains constant.
Consider an example, where the `volatile` keyword tells the compiler not to optimize the code by assuming that the value of variable won’t change. This is crucial in scenarios involving hardware registers, multi-threaded programs, or signal handlers, where variables may change due to external events.
Q29. Write a C program to print the Fibonacci series using recursion.
Ans) The Fibonacci series is a sequence of numbers where each number is the sum of the two previous ones, starting from 0 and 1. The sequence basically begins: 0, 1, 1, 2, 3, 5, 8, and so on. Here is a C program to print the Fibonacci series using recursion:
C Program For Interview Questions – Fibonacci Series using Recursion |
#include int fibonacci(int n) { if (n <= 1)
return n; else return fibonacci(n – 1) + fibonacci(n – 2); } int main() { int n, i; printf(“Enter the number of terms: “); scanf(“%d”, &n); printf(“Fibonacci Series: “); for (i = 0; i < n; i++) { printf(“%d “, fibonacci(i)); |
Output- Enter the number of terms: 10Fibonacci Series: 0 1 1 2 3 5 8 13 21 34 |
Learn C Programming With PW Skills
Are you looking to start your career as a software developer?
Enroll in our thefullstack programming powerhouse course to learn the basic and advanced concepts of all the in-demand programming languages, whether you are a beginner or an experienced programmer looking to polish your skills, enrolling in this course will help you learn all the essential concepts necessary for starting a career as a software developer.
The key features of this course that makes it a standout choice in the market include- Expert-led teaching sessions, PW lab for code practice, daily practice sheets for learning, regular doubt-clearing sessions, resume review sessions by experts, interview preparation sessions, alumni support, job placement assistance and much more!
So what are you waiting for, visit thefullstack.co.in today and grab your seat in this exciting journey.
C Program For Interview Questions FAQs
What is a null pointer in C?
A null pointer is a pointer that doesn’t point to any valid memory location. the pointer is not intended to point to any object.
What is the difference between == and = in C?
== is the equality operator used to compare two values, whereas = is the assignment operator used to assign a value to a variable.
How can you prevent memory leaks in C?
To prevent memory leaks in C programming language, ensure that every dynamically allocated memory using malloc(), calloc(), or realloc() is deallocated using the free() function when it is no longer needed.
Leave a Reply