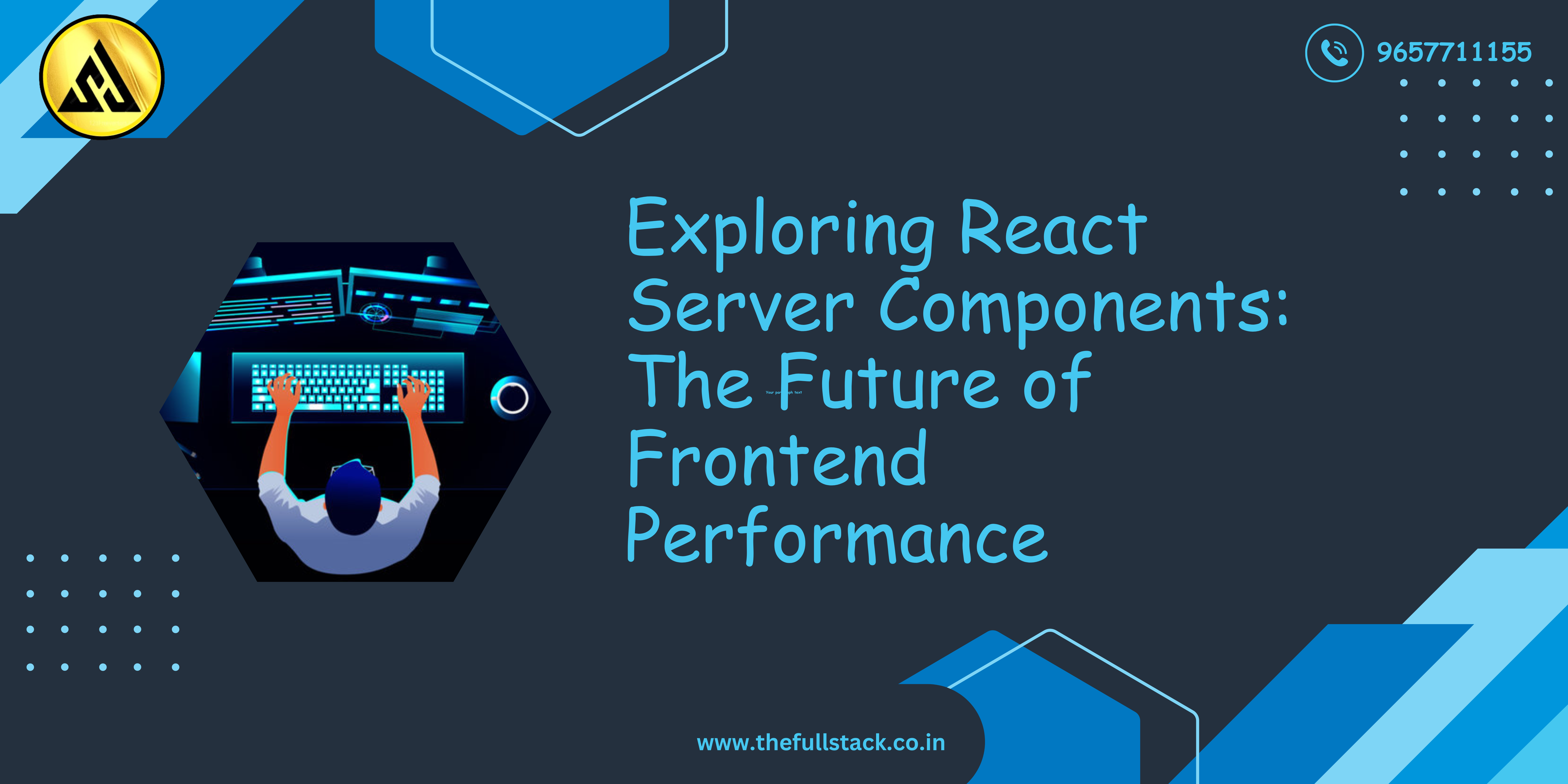
Exploring React Server Components: The Future of Frontend Performance
Introduction
One of Reacts most revolutionary developments is React Server Components (RSCs), which have been developed over time to maximize frontend performance. RSCs, which were first introduced in late 2020 and are now being progressively included into contemporary React apps, have the potential to completely transform the way we create effective, high-performing online applications. This article examines the foundations of React Server Components, their benefits, and how they will influence frontend development in the future.
What Are React Server Components?
React Server Components (RSCs) are a new type of component that runs exclusively on the server, allowing developers to offload expensive computations and data-fetching logic from the client side. Unlike traditional React components that bundle everything into the client-side JavaScript, RSCs enable zero client-side JavaScript overhead, leading to faster load times and improved performance.
Key Characteristics of RSCs:
- No Client-Side JavaScript Execution: Unlike standard React components, RSCs do not ship JavaScript to the browser.
- Improved Performance: Since components are processed on the server, they reduce the need for hydration and re-rendering on the client.
- Seamless Data Fetching: They allow developers to fetch data directly within the component, reducing the need for additional API calls on the client.
- Interoperability with Client Components: Developers can mix and match server and client components as needed.
Benefits of React Server Components
1. Reduced Bundle Size
Large JavaScript bundles are a major source of performance issues in React apps. By keeping unnecessary logic on the server, RSCs contribute to smaller client-side bundle sizes and quicker initial page loads.
2. Enhanced User Experience
By rendering components server-side and delivering fully rendered content to the client, RSCs minimize client-side computation, leading to a smoother user experience, especially on low-powered devices.
3. Optimized Data Fetching
Traditionally, React developers have relied on hooks like useEffect
to fetch data, which can lead to unnecessary API calls and waterfall requests. With RSCs, data fetching occurs on the server, eliminating extra network requests from the client.
4. SEO and Accessibility Benefits
Since React Server Components deliver pre-rendered HTML from the server, they enhance SEO rankings and improve accessibility for users who rely on screen readers.
How Do React Server Components Work?
Understanding the Component Hierarchy
React now categorizes components into three types:
- Server Components – Executed on the server, do not ship JavaScript to the client.
- Client Components – Executed in the browser, interact with user events.
- Shared Components – Can be used in both environments.
Example of a React Server Component:
// Server Component: Fetching Data on the Server
async function ProductList() {
const products = await fetch("https://api.example.com/products").then(res => res.json());
return (
<ul>
{products.map(product => (
<li key={product.id}>{product.name}</li>
))}
</ul>
);
}
export default ProductList;
Integrating React Server Components into Your App
1. Use a React Framework with RSC Support
To take full advantage of React Server Components, frameworks like Next.js 13+ (which fully supports RSCs) provide an optimized environment.
2. Distinguishing Server and Client Components
To use client-side interactivity, add 'use client'
at the top of a file to indicate a Client Component.
'use client';
function Button() {
return <button onClick={() => alert("Clicked!")}>Click Me</button>;
}
export default Button;
3. Mixing Server and Client Components
You can nest Client Components inside Server Components, but not vice versa.
import Button from "./Button";
async function ProductPage() {
const product = await fetch("https://api.example.com/product/1").then(res => res.json());
return (
<div>
<h1>{product.name}</h1>
<Button />
</div>
);
}
export default ProductPage;
The Future of Frontend Development with RSCs
React Server Components represent a major shift in how we think about frontend performance and user experience. As adoption increases, we can expect:
- More server-first React frameworks optimizing for performance.
- Less reliance on CSR (Client-Side Rendering) for data-heavy applications.
- Better developer experience with reduced complexity in data fetching and state management.
Conclusion
React Server Components are redefining frontend performance by reducing JavaScript bundle sizes, optimizing data fetching, and enhancing user experience. As the ecosystem evolves, developers who embrace RSCs will be at the forefront of building the next generation of fast, efficient web applications.
Are you excited about React Server Components? Share your thoughts in the comments!
It might be helpful for you:
Leave a Reply